Gist: Dictionary to CSV in Python
Use Python to create CSV files that play nicely with Excel.
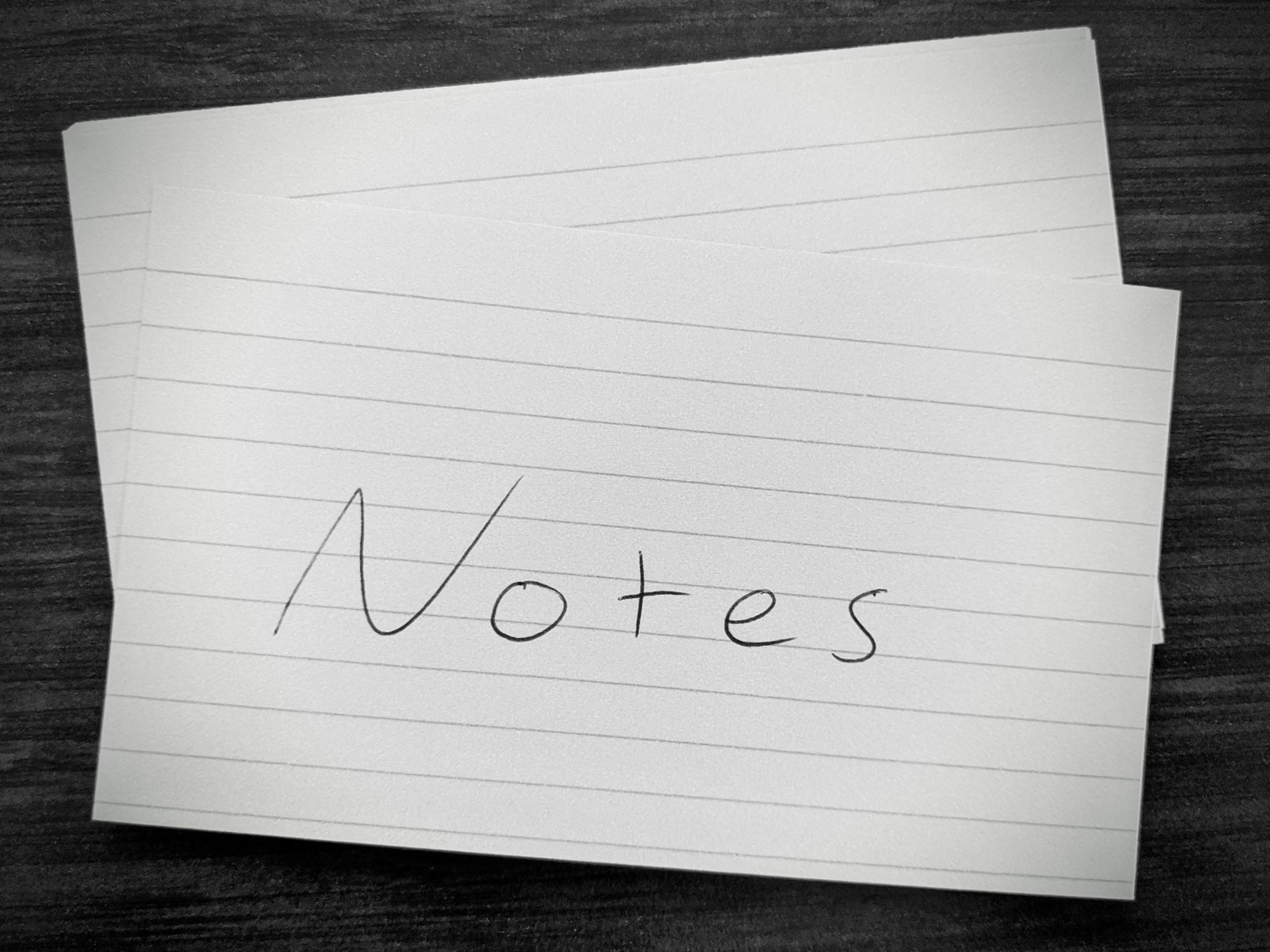
I noticed that the CSVs I created in Python didn't load properly when opened in Excel. Certain non-ASCII unicode characters were converted and displayed as different, incorrect characters. Additionally, improper character escaping meant that text fields containing newlines were sometimes split into different rows. Using the template below solves both of these issues.
import csv
arbitrary_list_of_dictionaries = [{'hello': 'world'}, {'hello': 'you'}]
arbitrary_output_path = 'output.csv'
# Use the keys from the first dictionary as field names in CSV
field_names = [key for key in arbitrary_list_of_dictionaries[0].keys()]
# Note: The 'encoding' argument is important. It adds a BOM (byte order mark)
# to the beginning of the file.
with open(arbitrary_output_path, 'w', encoding='utf-8-sig') as output_file:
# Note: The 'dialect' argument ensures that the outputted CSV matches
# Excel's specifications
writer = csv.DictWriter(file, fieldnames=field_names, dialect=csv.excel)
writer.writeheader()
for dictionary in arbitrary_list_of_dictionaries:
writer.writerow(dictionary)